
Audio Content Classification Tutorial: A Comprehensive Guide with Python Code
0
2
0
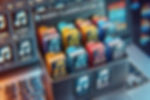
Introduction
Audio content classification, a prominent field in machine learning, involves training algorithms to recognize and categorize audio data into various classes or labels. This capability has a wide range of applications, including speech recognition, music genre classification, and environmental sound analysis. In this tutorial, we will delve into the fundamentals of audio content classification and provide you with a step-by-step guide, complete with Python code, to create your own audio classifier.
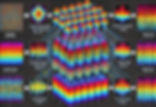
Understanding Audio Content Classification
Audio content classification is the process of assigning labels or categories to audio files based on their content. For example, classifying music tracks into genres, identifying spoken languages in speech recordings, or distinguishing between different types of environmental sounds are all examples of audio content classification.
Audio content classification involves teaching a machine learning model to recognize patterns in audio data and assign appropriate labels to them. This process usually consists of the following steps:
1. Data Collection and Preprocessing:Â
To build our audio content classifier, we need a labeled dataset containing audio clips of music and speech. You can create your own dataset or use publicly available datasets. Once you have your dataset, you'll need to preprocess the audio files. This includes converting audio files into a common format (e.g., WAV), extracting relevant features, and splitting the dataset into training and testing sets.
2. Feature Extraction:Â
Feature extraction is a crucial step in audio content classification. It involves transforming raw audio data into a format suitable for machine learning models. Common audio features include:
Mel-frequency cepstral coefficients (MFCCs):Â These represent the spectral characteristics of audio and are widely used in audio processing tasks.
Chroma feature:Â This captures the pitch content of audio.
Spectral contrast:Â It measures the difference in amplitude between peaks and valleys in the audio spectrum.
3. Model Selection:Â
Choosing the right machine learning or deep learning model is crucial for audio content classification. Popular options include:
Convolutional Neural Networks (CNNs): Effective for image and spectrogram-based audio classification.
Recurrent Neural Networks (RNNs): Suitable for sequential audio data, such as speech recognition.
Support Vector Machines (SVMs) and Random Forests: Traditional machine learning models that work well with extracted audio features.
Transfer Learning: You can use pre-trained models like VGGish (for audio) and fine-tune them for your specific classification task.
4. Model Training:Â
Now that we have our model architecture in place, we can proceed with training. Split your preprocessed dataset into training and testing sets, and train the model using the extracted audio features.
5. Model Evaluation:Â
Once the model is trained, it's essential to evaluate its performance. You can use metrics such as accuracy, precision, recall, and F1-score to assess how well your audio content classifier performs on the test set.
6. Fine-Tuning and Optimization
To improve your audio content classifier's performance, you can explore various techniques such as data augmentation, model ensembles, or more complex neural network architectures. Fine-tuning is an iterative process that often requires experimentation and patience.
7. Deployment
Once you are satisfied with your audio content classification model's performance, you can deploy it to make predictions on new audio data. This could involve integrating it into a mobile app, a web service, or an IoT device, depending on your application.
Creating Your Own Audio Content Classifier
In this section, we will guide you through the process of building an audio content classifier using Python. We'll use the TensorFlow and Keras libraries for constructing and training our model. Our example will focus on classifying music genres, but you can adapt the code for other audio classification tasks.
Step 1: Installing Dependencies
Before getting started, ensure you have the required libraries installed. Open your terminal and execute the following commands:
pip install tensorflow pip install librosa pip install numpy pip install pandas |
Step 2: Dataset Preparation
For our example, let's use the GTZAN dataset, which contains 10 music genres. You can download the dataset from here. Extract the dataset and organize it into separate folders for each genre.
Step 3: Feature Extraction
We'll use the Librosa library to extract Mel-frequency cepstral coefficients (MFCCs) from the audio clips. MFCCs capture the spectral characteristics of audio and are commonly used for audio analysis.
import librosa import numpy as np def extract_features(file_path): Â Â Â Â audio, sample_rate = librosa.load(file_path, res_type='kaiser_fast') Â Â Â Â mfccs = librosa.feature.mfcc(y=audio, sr=sample_rate, n_mfcc=13) Â Â Â Â return np.mean(mfccs, axis=1) |
Step 4: Data Preprocessing
Prepare your dataset by extracting features from each audio clip and organizing them along with their corresponding labels.
import os import pandas as pd data = {'filename': [], 'label': []} genres = ['blues', 'classical', 'country', 'disco', 'hiphop', 'jazz', 'metal', 'pop', 'reggae', 'rock'] for genre in genres: Â Â Â Â genre_folder = f'path/to/dataset/{genre}' Â Â Â Â for filename in os.listdir(genre_folder): Â Â Â Â Â Â Â Â if filename.endswith('.wav'): Â Â Â Â Â Â Â Â Â Â Â Â data['filename'].append(f'{genre_folder}/{filename}') Â Â Â Â Â Â Â Â Â Â Â Â data['label'].append(genre) df = pd.DataFrame(data) |
Step 5: Encoding Labels
Convert the genre labels into numerical representations using label encoding.
from sklearn.preprocessing import LabelEncoder label_encoder = LabelEncoder() df['label'] = label_encoder.fit_transform(df['label']) |
Step 6: Splitting the Dataset
Divide the dataset into training and testing sets.
from sklearn.model_selection import train_test_split X_train, X_test, y_train, y_test = train_test_split(df['filename'], df['label'], test_size=0.2, random_state=42) |
Step 7: Building the Model
Choose a suitable machine learning or deep learning model for your audio content classification task. In this case, Construct a simple Convolutional Neural Network (CNN) for audio classification.
import tensorflow as tf from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense, Dropout model = Sequential([ Â Â Â Â Conv2D(32, (3, 3), activation='relu', input_shape=input_shape), Â Â Â Â MaxPooling2D((2, 2)), Â Â Â Â Conv2D(64, (3, 3), activation='relu'), Â Â Â Â MaxPooling2D((2, 2)), Â Â Â Â Conv2D(128, (3, 3), activation='relu'), Â Â Â Â MaxPooling2D((2, 2)), Â Â Â Â Flatten(), Â Â Â Â Dense(128, activation='relu'), Â Â Â Â Dropout(0.3), Â Â Â Â Dense(num_classes, activation='softmax') ]) model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy']) |
Step 8: Model Training
Prepare the input data (audio features) and labels.
Train the selected model using the training dataset.
Monitor the training process using metrics like accuracy and loss.
batch_size = 32 epochs = 50 history = model.fit(X_train, y_train, batch_size=batch_size, epochs=epochs, validation_data=(X_test, y_test)) |
Step 9: Model Evaluation
Evaluate your trained model using the testing dataset. Metrics like accuracy, precision, recall, and F1-score can provide insights into the model's performance.
test_loss, test_acc = model.evaluate(X_test, y_test, verbose=2) print('\nTest accuracy:', test_acc) |
Step 10: Model Optimization
Fine-tune hyperparameters, experiment with different architectures, and consider techniques like regularization to improve your model's accuracy.
Step 11: Inference and Prediction:
Once your model is trained and optimized, use it to classify new, unseen audio samples.
Conclusion
In this tutorial, we've explored the intriguing domain of audio content classification using Python. By following the steps outlined above, you can now embark on your journey to automatically categorize audio data. Whether it's for music genres, speech recognition, or any other application, audio content classification holds immense potential.
Remember, this tutorial only scratches the surface of what's possible. You can enhance your models using deep learning techniques, try different classifiers, and experiment with various feature extraction methods. As you delve deeper into this field, you'll discover the power of Python in unlocking insights from audio data, revolutionizing the way we interact with and understand the auditory world around us.