
Basics of Deep Learning for Audio Applications: A Comprehensive Guide
0
7
0

Introduction
In the rapidly evolving landscape of artificial intelligence (AI), deep learning has emerged as a transformative technology with remarkable applications across various domains. One such domain that has witnessed significant advancements is audio processing. Deep learning techniques have revolutionized how we interact with audio, enabling tasks like speech recognition, music generation, and sound classification with unprecedented accuracy. In this article, we will delve into the basics of deep learning for audio applications, exploring key concepts, techniques, and even providing illustrative Python code examples.
Understanding Deep Learning for Audio
Deep learning is a subset of machine learning that involves training artificial neural networks to learn and make decisions from data. In the context of audio applications, deep learning algorithms can be designed to process, analyze, and generate audio signals. Some common audio tasks that deep learning can tackle include:
Speech Recognition: Converting spoken language into text.
Audio Classification: Categorizing audio into predefined classes (e.g., genre classification, sound event detection).
Sound Generation: Creating new audio samples, including music and speech synthesis.
Audio Enhancement: Removing noise, echoes, or other unwanted artifacts from audio signals.
Basics of Neural Networks
At the core of deep learning are neural networks. These are structures inspired by the human brain's interconnected neurons. Neural networks consist of layers of interconnected nodes (neurons) that process and transform data. The key layers in a neural network are:
Input Layer:Â This is where the raw audio data is fed into the network.
Hidden Layers:Â These layers process the data through mathematical transformations.
Output Layer:Â The final layer produces the desired output, such as recognizing a sound or classifying it into categories.
Training Deep Learning Models for Audio
Training deep learning models for audio applications involves several crucial steps:
Data Collection: Gathering a diverse and representative dataset is crucial. The dataset should include a variety of audio samples relevant to the task at hand.
Data Preprocessing: Preprocessing involves converting audio data into a suitable format, extracting features like spectrograms or MFCCs, and normalizing the data.
Model Architecture: Choosing the right deep learning architecture for the specific audio task is essential. As discussed earlier, CNNs, RNNs, LSTMs, or Transformer-based models may be suitable depending on the task.
Training: Training involves feeding the model with labeled data and adjusting its parameters (weights and biases) to minimize a loss function, making predictions closer to the ground truth labels.
Validation: After training, the model's performance is evaluated using a separate validation dataset to ensure it generalizes well to new, unseen data.
Fine-Tuning: Depending on the results, fine-tuning the model and hyperparameters may be necessary to improve performance.
Testing:Â Evaluate the model on a test dataset to measure its generalization ability.
Deployment: Once the model performs satisfactorily, deploy it in your application for real-time processing.
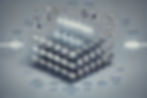
Audio Data Representation
Audio data is typically represented as a waveform, which is a plot of the amplitude of the sound signal against time. However, neural networks cannot directly work with raw waveforms due to their temporal nature. Instead, audio data is transformed into a more suitable format, such as a spectrogram or a mel spectrogram. A spectrogram is a visual representation that shows how the frequency content of a signal changes over time, while a mel spectrogram groups frequencies in a way that approximates human auditory perception. Python's libraries like Librosa and TensorFlow's tf.signal provide tools to compute spectrograms.
import librosa import librosa.display import matplotlib.pyplot as plt # Load audio file audio_path = 'path_to_audio_file.wav' y, sr = librosa.load(audio_path) # Compute spectrogram D = librosa.amplitude_to_db(np.abs(librosa.stft(y)), ref=np.max) plt.figure(figsize=(10, 6)) librosa.display.specshow(D, sr=sr, x_axis='time', y_axis='log') plt.colorbar(format='%+2.0f dB') plt.title('Spectrogram') plt.show() |
Building Deep Learning Models for Audio
1. Convolutional Neural Networks (CNNs) for Audio
Convolutional Neural Networks, well-known for their success in image processing tasks, can also be applied to audio data in the form of spectrograms. CNNs use convolutional layers to automatically learn hierarchical features from the data. In the case of audio, these layers can learn to detect relevant patterns in both time and frequency dimensions. Let's take a look at a simplified example of using a CNN for audio classification using the Keras library:
import tensorflow as tf from tensorflow.keras import layers, models # Build a simple 1D CNN model for audio classification model = models.Sequential() model.add(layers.Conv1D(32, 3, activation='relu', input_shape=input_shape)) model.add(layers.MaxPooling1D(2)) model.add(layers.Conv1D(64, 3, activation='relu')) model.add(layers.MaxPooling1D(2)) model.add(layers.Conv1D(128, 3, activation='relu')) model.add(layers.GlobalMaxPooling1D()) model.add(layers.Dense(num_classes, activation='softmax')) # Compile the model model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'] model.fit(X_train, y_train, validation_data=(X_test, y_test), epochs=10) |
2. Recurrent Neural Networks (RNNs) for Audio
Recurrent Neural Networks are particularly effective for tasks involving sequential data, such as speech recognition and music generation. Audio data is inherently sequential, as audio samples depend on their previous and future samples. RNNs utilize hidden states to capture temporal dependencies in data. Long Short-Term Memory (LSTM) networks, a type of RNN, are commonly used for audio processing due to their ability to retain information over longer sequences. Below is a simplified example of an audio generation model using LSTM:
# Build an LSTM model for audio sequence generation model = models.Sequential() model.add(layers.LSTM(128, return_sequences=True, input_shape=input_shape)) model.add(layers.LSTM(128, return_sequences=True)) model.add(layers.TimeDistributed(layers.Dense(num_classes, activation='softmax'))) # Compile the model model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy']) model.fit(X_train, y_train, validation_data=(X_test, y_test), epochs=10) |
3. Transfer Learning in Audio
Transfer learning involves leveraging pre-trained models on large datasets for specific tasks. This approach can be incredibly effective in audio applications where obtaining a large labeled dataset might be challenging. By fine-tuning pre-trained models like VGG16 or ResNet on smaller audio datasets, one can achieve remarkable results even with limited data.
from tensorflow.keras.applications import VGG16 # Load pre-trained VGG16 model (trained on ImageNet) base_model = VGG16(weights='imagenet', include_top=False, input_shape=input_shape) # Add custom layers for audio classification model = models.Sequential() model.add(base_model) model.add(layers.GlobalAveragePooling2D()) model.add(layers.Dense(num_classes, activation='softmax')) # Compile and fine-tune the model model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy']) model.fit(X_train, y_train, validation_data=(X_test, y_test), epochs=10) |
4. Generative Adversarial Networks (GANs) for Audio
Generative Adversarial Networks have opened doors to audio generation tasks. WaveGAN, for instance, generates realistic audio samples by training a generator to produce audio waveforms that are indistinguishable from real audio data. This is achieved through a game between the generator and a discriminator that evaluates the realism of generated audio.
# Example of using WaveGAN for audio generation import tensorflow as tf from tensorflow.keras.layers import Dense, Reshape, Conv1DTranspose generator = tf.keras.Sequential([ Â Â Â Â Dense(256, activation='relu', input_shape=(latent_dim,)), Â Â Â Â Reshape((1, 256)), Â Â Â Â Conv1DTranspose(128, 25, strides=4, activation='relu'), Â Â Â Â Conv1DTranspose(64, 25, strides=4, activation='relu'), Â Â Â Â Conv1DTranspose(1, 25, strides=4, activation='tanh') ]) |
Applications of Deep Learning in Audio
Deep learning has opened up numerous possibilities in the field of audio processing. Here are some common applications:
1. Speech Recognition
Deep learning models, especially recurrent neural networks, have significantly advanced automatic speech recognition (ASR) systems. These systems can convert spoken language into text and find applications in voice assistants, transcription services, and more.
2. Music Classification and Recommendation
Deep learning algorithms are used to classify music into genres and create personalized music recommendations for users. These models analyze audio features to make accurate music recommendations based on user preferences.
3. Noise Reduction
In audio processing, eliminating background noise while preserving the clarity of the desired sound is a challenging task. Deep learning models, such as denoising autoencoders and convolutional neural networks, excel at noise reduction. They can distinguish between signal and noise components in audio and suppress unwanted sounds effectively.
4. Voice Synthesis
Deep learning has enabled the development of highly realistic text-to-speech (TTS) systems that can convert text into natural-sounding speech. These systems have applications in voice assistants, audiobooks, and accessibility tools.
5. Music Generation
Generative models like Variational Autoencoders (VAEs) and Generative Adversarial Networks (GANs) can generate music compositions or imitate the style of famous composers. These models have the potential to revolutionize the music industry.
6. Speaker Identification
Deep learning techniques are used in speaker identification systems to recognize and verify the identity of individuals based on their voice. This has applications in security, voice assistants, and more.
Challenges in Deep Learning for Audio
While deep learning has shown remarkable success in audio applications, it also comes with its challenges:
1. Data Size and Quality
Training deep learning models for audio often requires large and high-quality datasets. Collecting and annotating such datasets can be time-consuming and costly.
2. Computational Resources
Deep learning models, especially deep neural networks, demand significant computational resources for training and inference. Specialized hardware like Graphics Processing Units (GPUs) or TPUs is often required.
3. Interpretability
Deep learning models can be challenging to interpret, making it difficult to understand why a model makes a particular prediction or decision, which is crucial for some applications, such as medical diagnosis.
4. Overfitting
Deep neural networks are prone to overfitting, where the model performs well on the training data but poorly on unseen data. Techniques like dropout and regularization are used to mitigate this issue.
Conclusion
In the realm of audio applications, deep learning offers a multitude of possibilities, from speech recognition to music composition. Through spectrogram-based data representation, Convolutional and Recurrent Neural Networks, and the power of transfer learning, developers and researchers can tackle intricate audio tasks with unprecedented accuracy. As the field of deep learning continues to evolve, we can expect even more sophisticated techniques to emerge, further pushing the boundaries of what's achievable in audio processing.
Remember, mastering deep learning for audio applications takes practice and experimentation. Feel free to explore the provided code examples and adapt them to your specific use cases. With dedication and creativity, you can contribute to the exciting advancements in this dynamic field.