
The rapid evolution of technology has ushered in a new era of machine learning workflows, with deep learning taking center stage. Thanks to advancements in parallel computing and supporting tools, intricate and deep neural networks, once considered impractical, have become feasible and effective.
The availability of powerful libraries like Tensorflow, Torch, and Deeplearning4j has democratized deep learning development, making it accessible beyond academia and large tech companies. Notably, industry giants such as Huawei and Apple have integrated specialized deep learning processors into their latest devices to drive deep learning applications.
Across diverse domains, deep learning has showcased its prowess. Google's AlphaGo achieved the unthinkable by defeating human players in the ancient and complex game of Go. Sony's Flow Machines project birthed a neural network that composes music in the style of renowned musicians. Apple's FaceID leverages deep learning to recognize and track changes in users' faces over time.
In this article, we delve into the intersection of deep learning, natural language processing (NLP), and the world of wine. We'll guide you through building a deep learning model capable of deciphering expert-written natural language wine reviews and deducing the wine variety they pertain to.
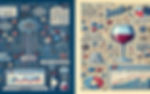
Text Classification
At its core, text classification is a form of machine learning that involves training algorithms to automatically assign predefined categories or labels to text documents based on their content. It's akin to teaching a machine to distinguish between different types of text by learning patterns and relationships from labeled examples. These labeled examples, often referred to as the training dataset, fuel the algorithm's learning process. The ultimate goal is to enable the algorithm to accurately predict the category of unseen text.

The Power of Deep Learning in NLP
The integration of deep learning and natural language processing has yielded remarkable results. Deep learning excels in capturing intricate sentence structures and semantic relationships between words. For instance, the cutting-edge sentiment analysis models employ deep learning to understand complex linguistic nuances like negations and mixed sentiments.
The advantages of deep learning for NLP are manifold:
Flexibility in Model Design: Deep learning models offer remarkable flexibility, allowing for experimentation with various structures by adding or removing layers as needed. This adaptability extends to building models with diverse outputs, pivotal for understanding complex linguistic structures and developing applications like translations, chatbots, and text-to-speech systems.
Reduced Domain Knowledge Requirement: While some domain knowledge is essential for creating effective deep learning models, these algorithms can autonomously learn feature hierarchies. This mitigates the need for exhaustive domain expertise in developing deep learning-based NLP algorithms, a notable advantage in the intricate realm of natural language.
Seamless Continual Learning: Updating deep learning algorithms with new data is straightforward, particularly valuable for dynamic datasets. Some machine learning methods necessitate running the entire dataset through the model for updates, posing challenges with live, large datasets.
The Challenge at Hand
Our focus lies in constructing a deep learning algorithm to predict the wine variety based on review text. We'll employ the wine magazine dataset available at Kaggle.
Can our neural network recognize this description as belonging to a white blend? While wine connoisseurs might detect white wine attributes like apple, citrus, and acidity, can we train our model to discern such subtleties? Furthermore, can it distinguish between a white blend review and a pinot grigio review?
Similar Algorithms as Baselines
Our task resembles an NLP classification problem. Other NLP classification algorithms, such as naive Bayes and support vector machines (SVM), have found success in various applications. For example, naive Bayes has been used for spam detection, and SVMs have classified healthcare progress notes. Implementing simplified versions of these algorithms provides a baseline for our deep learning model.

1. Importing Libraries
Let's start by importing the necessary libraries:
import numpy as np from sklearn.naive_bayes import MultinomialNB from sklearn.feature_extraction.text import CountVectorizer import pandas as pd from collections import Counter from sklearn.model_selection import train_test_split from sklearn.feature_extraction.text import TfidfTransformer |
2. Loading and Preprocessing Data
Load your dataset using Pandas and perform basic preprocessing steps such as removing duplicates and handling missing values:
df = pd.read_csv('your_dataset.csv')Â # Load your dataset df.drop_duplicates(inplace=True) df.dropna(inplace=True) |
3. Feature Extraction
Convert the cleaned text into numerical features using techniques like Count Vectorization:
df = pd.read_csv('data/wine_data.csv') counter = Counter(df['variety'].tolist()) top_10_varieties = {i[0]: idx for idx, i in enumerate(counter.most_common(10))} df = df[df['variety'].map(lambda x: x in top_10_varieties)] description_list = df['description'].tolist() varietal_list = [top_10_varieties[i] for i in df['variety'].tolist()] varietal_list = np.array(varietal_list) count_vect = CountVectorizer() x_train_counts = count_vect.fit_transform(description_list) tfidf_transformer = TfidfTransformer() x_train_tfidf = tfidf_transformer.fit_transform(x_train_counts) |
4. Splitting the Dataset
Split the dataset into training and testing sets:
train_x, test_x, train_y, test_y = train_test_split(x_train_tfidf, varietal_list, test_size=0.3) |
5. Building the Classification Model
Train a classification model using the training data:
Naive Bayes
Naive Bayes for NLP involves preprocessing text using TF-IDF and then running multinomial naive Bayes on the preprocessed data. This approach focuses on prominent words within a document. Implementing naive Bayes might look like this:
clf = MultinomialNB().fit(train_x, train_y) |
Support Vector Machine
Alternatively, we can use a support vector machine to classify our data. By replacing the classifier definition and running the code, we achieve:
clf = SVC(kernel='linear').fit(train_x, train_y) |
6. Evaluating the Model
Evaluate the model's performance on the testing data:
y_score = clf.predict(test_x) n_right = 0 for i in range(len(y_score)): Â Â Â Â if y_score[i] == test_y[i]: Â Â Â Â Â Â Â Â n_right += 1 print("Accuracy: %.2f%%" % ((n_right/float(len(test_y)) * 100))) |
Building the Deep Learning Model
In this section, we'll leverage Keras with Tensorflow to construct our deep learning model. Keras, a user-friendly Python library, simplifies model building compared to the lower-level Tensorflow API. Our model will encompass an embedding layer, a convolutional layer, and a dense layer to extract semantic information and structural patterns from the data.
Data Preprocessing
We begin by restructuring the data to facilitate neural network processing. We'll replace words with unique numbers, combined with embedding vectors for flexibility and semantic sensitivity. In practice, prioritizing common words and excluding frequently used ones (e.g., articles) would enhance preprocessing efficiency.
from nltk import word_tokenize from collections import defaultdict def count_top_x_words(corpus, top_x, skip_top_n):     count = defaultdict(lambda: 0)     for c in corpus:         for w in word_tokenize(c):             count[w] += 1     count_tuples = sorted([(w, c) for w, c in count.items()], key=lambda x: x[1], reverse=True)     return [i[0] for i in count_tuples[skip_top_n: skip_top_n + top_x]] def replace_top_x_words_with_vectors(corpus, top_x):     topx_dict = {top_x[i]: i for i in range(len(top_x))}     return [         [topx_dict[w] for w in word_tokenize(s) if w in topx_dict]         for s in corpus     ], topx_dict def filter_to_top_x(corpus, n_top, skip_n_top=0):     top_x = count_top_x_words(corpus, n_top, skip_n_top)     return replace_top_x_words_with_vectors(corpus, top_x) |
Model Construction
With Keras, constructing the model is straightforward. We'll utilize an embedding layer, a convolutional layer, and a dense layer to harness deep learning's capabilities for our application.
from keras.models import Sequential from keras.layers import Dense, Conv1D, Flatten from keras.layers.embeddings import Embedding from keras.preprocessing import sequence from keras.utils import to_categorical import pandas as pd from collections import Counter from sklearn.model_selection import train_test_split from lib.get_top_xwords import filter_to_top_x df = pd.read_csv('data/wine_data.csv') counter = Counter(df['variety'].tolist()) top_10_varieties = {i[0]: idx for idx, i in enumerate(counter.most_common(10))} df = df[df['variety'].map(lambda x: x in top_10_varieties)] description_list = df['description'].tolist() mapped_list, word_list = filter_to_top_x(description_list, 2500, 10) varietal_list_o = [top_10_varieties[i] for i in df['variety'].tolist()] varietal_list = to_categorical(varietal_list_o) max_review_length = 150 mapped_list = sequence.pad_sequences(mapped_list, maxlen=max_review_length) train_x, test_x, train_y, test_y = train_test_split(mapped_list, varietal_list, test_size=0.3) max_review_length = 150 embedding_vector_length = 64 model = Sequential() model.add(Embedding(2500, embedding_vector_length, input_length=max_review_length)) model.add(Conv1D(50, 5)) model.add(Flatten()) model.add(Dense(100, activation='relu')) model.add(Dense(max(varietal_list_o) + 1, activation='softmax')) model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy']) model.fit(train_x, train_y, epochs=3, batch_size=64) y_score = model.predict(test_x) y_score = [[1 if i == max(sc) else 0 for i in sc] for sc in y_score] n_right = 0 for i in range(len(y_score)): Â Â Â Â if all(y_score[i][j] == test_y[i][j] for j in range(len(y_score[i]))): Â Â Â Â Â Â Â Â n_right += 1 print("Accuracy: %.2f%%" % ((n_right/float(len(test_y)) * 100))) |
Use Cases of Text Classification
1. Sentiment Analysis
Perhaps one of the most recognizable applications of text classification is sentiment analysis. It involves determining the sentiment or emotion expressed in a piece of text, whether it's positive, negative, or neutral. Businesses can employ sentiment analysis to gauge customer opinions about their products or services, enabling them to make informed decisions and enhance customer satisfaction.

2. Spam Detection
In the war against unwanted emails, text classification emerges as a champion. By training algorithms to differentiate between legitimate messages and spam, email providers can automatically filter out spam emails, ensuring that users only see relevant content in their inboxes.

3. Topic Categorization
Text classification plays a pivotal role in organizing vast collections of documents or articles into meaningful categories. News websites, for instance, can employ topic categorization to automatically classify news articles into sections such as politics, sports, technology, and more.
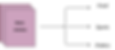
4. Language Identification
In our interconnected world, where content flows across language barriers, text classification can be used to automatically identify the language of a given text. This is invaluable for search engines, translation services, and content localization efforts.

5. Intent Recognition
Virtual assistants and chatbots rely heavily on text classification to understand user intent. By deciphering user inputs and classifying them into specific intents, these AI-powered systems can provide accurate responses and enhance user experiences.

6. Medical Diagnosis
In the medical field, text classification aids in diagnosing diseases from medical reports and patient records. Algorithms can predict medical conditions, reducing the time and effort required for diagnosis.
7. Financial Analysis
Financial institutions use text classification to analyze news articles, reports, and social media data for insights into market trends, investor sentiment, and financial events that may impact trading decisions.
Applications of Text Classification
1. Social Media Monitoring
Text classification aids companies in monitoring social media platforms. By classifying social media posts and comments, businesses can gain insights into customer opinions, trends, and emerging issues, allowing them to tailor their strategies accordingly.
2. Legal Document Analysis
Law firms and legal professionals harness text classification to analyze and categorize legal documents. This streamlines the process of information retrieval, case management, and legal research.
3. Medical Diagnostics
In the medical domain, text classification contributes to the analysis of medical records, patient notes, and research articles. It aids in disease classification, patient diagnosis, and drug discovery.
4. Customer Support Automation
Text classification enables automation of customer support processes. By categorizing customer queries, companies can provide quick and accurate responses, enhancing customer satisfaction and reducing response times.
5. Market Research
Businesses delve into market research by analyzing customer feedback, reviews, and survey responses. Text classification allows them to distill valuable insights from these unstructured textual sources.
6. E-commerce
Text classification enables e-commerce platforms to automatically categorize products, extract product attributes, and improve search functionalities, enhancing user experience.
7. Education
In the education sector, text classification assists in automating tasks such as grading student assignments, categorizing educational resources, and analyzing educational data for insights.
Conclusion
In this exploration of deep learning's role in NLP, we embarked on a journey to construct a classification model for analyzing wine reviews. Our deep learning model showcased its ability to rival and even surpass traditional machine learning algorithms. This serves as a testament to the efficacy of deep learning in replicating and enhancing results produced by domain-expertise-driven models.
As you journey deeper into the realm of deep learning and NLP, you'll encounter intricate techniques, complex architectures, and a universe of applications. This article forms a steppingstone, empowering you to unlock the potential of deep learning in deciphering the intricate tapestry of natural language and its vast applications.