
Exploring Emotion Analysis in Speech: Unveiling APIs for Emotional Insights
1
5
0
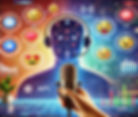
Introduction
The power of speech lies not only in the words spoken but also in the emotions conveyed. In today's digital era, understanding human emotions in speech has become a crucial aspect of various industries, ranging from customer service and mental health to entertainment and marketing. Fortunately, technology has evolved to a point where we can harness the capabilities of Application Programming Interfaces (APIs) to analyze emotions in speech. In this comprehensive guide, we'll delve into the realm of emotion analysis in speech and explore APIs that offer this capability, allowing us to tap into the nuances of human expression.
Understanding Emotion Analysis in Speech
Emotion analysis, also known as sentiment analysis, involves the detection and interpretation of human emotions from textual or verbal data. When applied to speech, it focuses on extracting emotional cues, such as joy, sadness, anger, and fear, from spoken words. This technology employs advanced natural language processing (NLP) and machine learning techniques to analyze speech patterns, tones, and linguistic cues that indicate emotional states.
The Importance of Emotion Analysis in Speech
Emotion analysis in speech involves the use of computational techniques to identify and categorize emotions expressed through vocal cues. This technology has wide-ranging applications:
Customer Service:Â Call centers and customer support can benefit from real-time emotion analysis to enhance customer interactions. Identifying customer frustration can prompt immediate assistance or tailored responses.
Healthcare:Â Mental health professionals can utilize emotion analysis to monitor and assess patients' emotional well-being remotely, providing timely interventions if necessary.
Education:Â Emotion analysis can be integrated into e-learning platforms to gauge students' engagement levels and adapt content to their emotional states.
Media and Entertainment:Â Emotion analysis can gauge audience reactions to movies, TV shows, and advertisements, aiding in content creation and marketing strategies.
APIs for Emotion Analysis in Speech
Now, let's explore some prominent APIs that offer emotion analysis in speech, enabling us to tap into the realm of human emotions.
1. Google Cloud Natural Language API:
Google's Cloud Natural Language API provides sentiment analysis for text and speech, offering the ability to identify emotions within spoken content. By analyzing text or audio input, this API can detect emotions such as joy, sorrow, anger, and surprise.
2. Microsoft Azure Cognitive Services - Speech SDK:
Microsoft's Azure Cognitive Services offers a Speech SDK that provides emotion analysis capabilities. Using this SDK, developers can integrate real-time emotion detection into their applications, opening avenues for emotionally aware interactions.
3. IBM Watson Tone Analyzer:
IBM's Watson Tone Analyzer is a versatile tool that can analyze emotions and tones within written text and transcribed speech. It categorizes emotions into dimensions like joy, sadness, fear, and anger, enabling a comprehensive understanding of emotional nuances.
4. Amazon Comprehend:
Amazon Comprehend offers sentiment analysis for text and speech, providing insights into the emotions behind spoken words. This API can be seamlessly integrated into applications, facilitating emotion-based interactions and experiences.
5. IBM Watson Speech to Text with Emotion Analysis:
IBM Watson's Speech to Text service provides an emotion analysis feature that can identify a range of emotions, such as joy, sadness, anger, and more, from spoken content. By utilizing this API, developers can extract valuable emotional insights from recorded speech data.
6. Affectiva Emotion AI:
Affectiva offers an Emotion AI API that specializes in analyzing emotions from facial expressions and vocal intonations. This API is particularly suitable for applications that require a multi-modal approach, combining facial and vocal emotion analysis
7. Microsoft Azure Text Analytics API
Microsoft's Azure Text Analytics API offers sentiment analysis, which evaluates text data (including speech transcriptions) to determine emotional sentiment. It assigns sentiment scores ranging from negative to neutral to positive, helping to gauge the emotional tone of speech.
The API employs machine learning models to recognize nuances in language, including sarcasm and idiomatic expressions, enhancing the accuracy of emotion analysis. It's a versatile tool suitable for applications ranging from customer feedback analysis to social media sentiment monitoring.
8. IBM Watson Natural Language Understanding API
IBM's Watson Natural Language Understanding API is another powerful tool for emotion analysis in speech. It can extract emotions such as joy, sadness, anger, fear, and disgust from text and speech data.
The API leverages advanced linguistic and psychological theories to provide nuanced emotional insights. It's particularly valuable for applications in mental health, as it can aid in assessing patients' emotional well-being through their speech patterns.
Implementing Emotion Analysis Using IBM Watson Speech to Text API
Let's explore how you can use the IBM Watson Speech to Text API for emotion analysis in speech.
Step 1: Set Up API Credentials
First, sign up for IBM Watson services and create credentials for the Speech to Text service. This will provide you with the API key and URL required to access the service.
Step 2: Install Required Libraries
You'll need the ibm-watson Python library to interact with the API.
pip install ibm-watson |
Step 3: Analyze Emotions
from ibm_watson import SpeechToTextV1 from ibm_cloud_sdk_core.authenticators import IAMAuthenticator # Set up authentication authenticator = IAMAuthenticator('YOUR_API_KEY') speech_to_text = SpeechToTextV1(authenticator=authenticator) speech_to_text.set_service_url('YOUR_SERVICE_URL') # Transcribe audio and analyze emotions with open('audio_file.wav', 'rb') as audio_file:     result = speech_to_text.recognize(         audio=audio_file,         content_type='audio/wav',         model='en-US_NarrowbandModel',         word_alternatives_threshold=0.9,         keywords=['colorado', 'tornado', 'tornadoes'],         keywords_threshold=0.5,         emotion=True # Enable emotion analysis     ).get_result() # Extract emotions from the result emotions = result['results'][0]['alternatives'][0]['emotion'] print(emotions) |
Implementing Emotion Analysis with Google Cloud Natural Language API
Now, let's walk through the process of implementing emotion analysis in speech using Python and Google Cloud Natural Language API.
1. Set Up Google Cloud Account:
Sign up for a Google Cloud account (https://cloud.google.com/).
Create a project and enable the Natural Language API.
2. Install Required Libraries:
Open your terminal and install the required libraries using the following commands:
pip install google-cloud-language pip install playsound |
3. Write Python Code:
import os from google.cloud import language_v1 # Set up Google Cloud credentials os.environ["GOOGLE_APPLICATION_CREDENTIALS"] = "path_to_your_service_account_key.json" # Initialize the client client = language_v1.LanguageServiceClient() # Specify the audio file path audio_path = 'path_to_audio_file.wav' # Read audio file as binary with open(audio_path, 'rb') as audio_file: Â Â Â Â content = audio_file.read() # Perform sentiment analysis document = language_v1.Document(content=content, type_=language_v1.Document.Type.PLAIN_TEXT) response = client.analyze_sentiment(request={'document': document}) # Get sentiment score and magnitude sentiment = response.document_sentiment score = sentiment.score magnitude = sentiment.magnitude # Determine emotion based on sentiment score if score > 0.2: Â Â Â Â emotion = "positive" elif score < -0.2: Â Â Â Â emotion = "negative" else: Â Â Â Â emotion = "neutral" # Print results print(f"Emotion: {emotion}") print(f"Sentiment Score: {score}") print(f"Sentiment Magnitude: {magnitude}") |
Implementing Emotion Analysis using the Microsoft Azure Text Analytics APIÂ
Let's explore a basic implementation of emotion analysis using the Microsoft Azure Text Analytics API in Python:
Import Required Libraries:
import requests # Replace with your Azure subscription key and endpoint subscription_key = 'YOUR_SUBSCRIPTION_KEY' endpoint = 'YOUR_ENDPOINT' |
Make API Request:
def analyze_emotion(text):     base_url = f'{endpoint}/text/analytics/v3.1/sentiment'     headers = {         'Content-Type': 'application/json',         'Ocp-Apim-Subscription-Key': subscription_key     }     data = {         'documents': [             {'id': '1', 'language': 'en', 'text': text}         ]     }     response = requests.post(base_url, json=data, headers=headers)     return response.json()['documents'][0]['sentiment'] |
Analyze Emotion:
text = "I'm really excited about this new project!" emotion = analyze_emotion(text) print(f'Emotion: {emotion}') |
Choosing the Right API
The choice of which emotion analysis API to use depends on your specific requirements, budget, and preferred cloud provider. All three options discussed here are capable of detecting emotions in speech, but they may differ in pricing, ease of use, and additional features. It's advisable to review the documentation and pricing details of each API to make an informed decision.
Building Your Own Emotion Analysis Model
While APIs offer a convenient way to perform emotion analysis in speech, you may want to build your own custom models for more specific requirements. Here's a high-level overview of the steps involved:
Data Collection:Â Gather a diverse dataset of spoken words or phrases annotated with their corresponding emotions.
Feature Extraction:Â Extract relevant features from the audio, such as Mel-frequency cepstral coefficients (MFCCs) or spectrograms.
Model Training:Â Train a machine learning model (e.g., deep neural network) on the extracted features, using the annotated dataset to predict emotions.
Evaluation:Â Assess the model's performance using metrics like accuracy, F1-score, and confusion matrices.
Deployment:Â Deploy the trained model as part of your application, making it available for real-time emotion analysis.
Continuous Improvement:Â Periodically update and fine-tune your model with new data to improve accuracy and adapt to changing speech patterns.
Building a custom model can be a complex task, and it requires a substantial amount of data and computational resources. However, it provides greater flexibility and control over your emotion analysis system.
Conclusion
As technology continues to advance, our ability to understand and analyze human emotions through speech becomes increasingly sophisticated. The APIs mentioned in this guide open up a world of possibilities for industries seeking to harness the power of emotional insights. By utilizing these APIs and implementing emotion analysis in speech through Python, we can enhance customer experiences, improve mental health diagnosis, enrich entertainment content, and gather valuable market research data. The fusion of technology and human emotion brings us closer to a future where our interactions with machines are not only functional but also empathetic and emotionally attuned.