
Exploring Zero-shot Text Classification with Hugging Face on Gradient Notebook
0
1
0
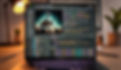
In the realm of Machine Learning, Zero-shot learning (ZSL) has emerged as a powerful paradigm, allowing models to classify data into classes that were not present in their training data. This concept mirrors how humans extrapolate knowledge from existing information to new concepts. ZSL is particularly valuable when obtaining labeled data for specific classes is time-consuming or expensive. One prominent application of ZSL is in text classification, where models can categorize text into predefined groups without explicit training for each category.
Hugging Face, an open-source machine learning platform, has gained widespread recognition, especially in Natural Language Processing (NLP). The platform provides an array of tools, including the Transformers library, which facilitates easy access to state-of-the-art NLP models. In this tutorial, we will explore the zero-shot text classification pipeline provided by Hugging Face and implement it using a Gradient Notebook, an accessible web-based Jupyter IDE with free GPUs.
Understanding Zero-shot Text Classification
Zero-shot text classification involves assigning predefined categories to input text without the need for a dedicated model trained on a specific dataset. This paradigm is particularly useful when labeled data is scarce or unavailable. It allows models to extrapolate knowledge from existing information, a strategy akin to how humans adapt their learning to new concepts based on prior knowledge. Applications of zero-shot learning span diverse domains, including Text Classification, Image Classification, Text-to-Image Generation, and Speech Translation.
In the context of text classification, the task involves categorizing text snippets into predefined classes. Traditional text classification is performed through supervised learning, where a model learns a mapping from input samples to class labels. Zero-shot text classification extends this concept by enabling the model to classify text into predefined classes without explicit training on labeled data.
Unveiling Hugging Face and Gradient Notebook
Before diving into the specifics, let's familiarize ourselves with Hugging Face and Gradient Notebook. Hugging Face is renowned for its open-source machine learning technologies, gaining popularity for its Transformers support. Transformers provide an accessible way to download, train, and infer state-of-the-art Natural Language Processing (NLP) models. On the other hand, Gradient Notebook is a web-based Jupyter IDE with free GPU access, enabling seamless development and collaboration for machine learning projects.
In this tutorial, we'll explore zero-shot text classification using Hugging Face's pipeline capabilities on Gradient Notebook. We'll not only walk through the implementation steps but also gain insights into the underlying mechanisms of the algorithm.
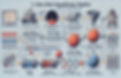
Prerequisites
Before we dive into the code, make sure you have the following prerequisites:
Python (3.6 or higher) installed.
An account on Hugging Face's Model Hub (https://huggingface.co/).
Access to the Gradient platform (https://gradient.paperspace.com/).
Setting Up Your Environment
Let's begin by installing the "transformers" library:
# Install Hugging Face Transformers and Gradient libraries !pip install transformers !pip install gradient # Import required libraries import transformers from transformers import pipeline |
Using Hugging Face Transformers for Zero-shot Text Classification
Hugging Face simplifies model inference through pipelines, abstracting complex code. We'll leverage this concept for zero-shot classification. The "Pipeline" class serves as the base for task-specific pipelines. In our case, the task is "zero-shot-classification," which triggers a child pipeline dedicated to zero-shot classification. Hugging Face offers various task-specific pipelines, each optimized for its task.
Next, we'll import the pipeline, define the task ("zero-shot-classification"), specify the device (GPU or CPU), and select an underlying model that supports the task:
from transformers import pipeline classifier = pipeline(     task="zero-shot-classification",     device=0, # Use GPU (device=0); CPU is device=-1 ) |
Performing Zero-shot Text Classification
With our classifier set up, let's explore some zero-shot classification examples. We'll provide a text snippet and a set of candidate labels. The model will predict the most relevant label for the text:
i# Text to classify text = "The Mars rover Opportunity has made some fascinating discoveries about the Red Planet." # Candidate class names or labels candidate_labels = ["Space Exploration", "Geology", "Astronomy", "Technology"] # Perform zero-shot text classification result = classifier(text, candidate_labels) # Print the result print(result) |
In this example, the classifier pipeline takes the input text and a list of candidate class labels. It returns a dictionary containing the labels and their corresponding scores for the given text. The label with the highest score is the predicted class.
Customizing Zero-shot Classification Templates
You can customize the zero-shot text classification by adjusting the model and tokenizer parameters of the pipeline function. For example, you can choose different pre-trained models or tokenizers based on your specific requirements.
# Customize the model and tokenizer custom_classifier = pipeline("zero-shot-classification", model="joeddav/xlm-roberta-large-xnli", tokenizer="joeddav/xlm-roberta-large-xnli") # Perform zero-shot text classification with custom model and tokenizer result = custom_classifier(text, candidate_labels) # Print the result print(result) |
Handling Multi-label Classification
In some cases, you might want to perform multi-label zero-shot text classification, where a single text document can be assigned multiple labels. You can achieve this by specifying the multi_label parameter as True.
# Create a multi-label zero-shot text classification pipeline multi_label_classifier = pipeline("zero-shot-classification", multi_label=True) # Perform multi-label zero-shot text classification result = multi_label_classifier(text, candidate_labels) # Print the result print(result) |
Going Under the Hood
Wondering how this magic works under the hood? The pipeline follows a sequence of steps to perform zero-shot classification:
Pre-Processing: Preprocessing involves a series of tasks to clean and normalize raw text data, including steps like removing punctuation, converting to lowercase etc . These preparatory steps ensure that text data is in a suitable format for subsequent model.
Tokenization: The input text is tokenized, with special tokens like [SEP] and [CLS] added as needed by the model.
Inference: The tokenized sequence is passed through the pre-trained model, which produces a softmax distribution across the candidate label set.
Post-processing: Depending on the model's output, post-processing may involve tasks like removing special tokens and trimming the output to a specific length.
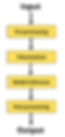
Concluding Thoughts
Zero-shot text classification is a powerful technique that allows you to classify text into categories that were not present in your training data. With the Hugging Face Transformers library and the Gradient platform, you can easily implement zero-shot text classification in your NLP projects. Experiment with different models and customizations to achieve the best results for your specific tasks. Happy classifying!
In this article, we discussed the concept of zero-shot text classification, its importance, and how to implement it using the Hugging Face Transformers library on Gradient. We covered setting up the environment, creating a zero-shot text classification pipeline, performing classification, customizing the pipeline, and handling multi-label classification. Zero-shot text classification opens up exciting possibilities in NLP, allowing you to classify text into categories that were never seen during training, and it can be a valuable tool in various applications.