
How to Implement Speech Recognition in Python: A Comprehensive Guide
0
33
0
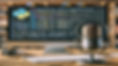
In the ever-evolving landscape of technology, speech recognition has emerged as a powerful tool with a wide range of applications. From voice assistants like Siri and Alexa to transcription services and language learning apps, speech recognition has revolutionized how humans interact with computers. Python, a popular programming language, offers a variety of libraries and tools that make implementing speech recognition a straightforward process. In this article, we will explore the steps to implement speech recognition in Python and discuss some key libraries that can help you achieve this seamlessly.
Understanding Speech Recognition
Speech recognition is the process of converting spoken language into written text. This technology has revolutionized human-computer interaction, enabling devices and applications to understand and respond to spoken commands. Whether it's voice assistants like Siri and Alexa, or transcription services that convert audio recordings into text, speech recognition has become an integral part of modern computing.
Getting Started with Speech Recognition in Python
Python is a popular programming language known for its simplicity and readability, making it an excellent choice for implementing speech recognition. Several libraries and APIs are available in Python that make it relatively easy to integrate speech recognition into your projects. Here are some reasons to consider implementing speech recognition in Python:
Abundance of Libraries:Â Python has a rich ecosystem of libraries and frameworks that simplify the implementation of speech recognition. You can choose from a variety of options based on your specific needs.
Cross-Platform Compatibility:Â Python runs on multiple platforms, including Windows, macOS, and Linux. This cross-platform compatibility ensures that your speech recognition application can be used by a broad audience.
Community Support:Â Python has a large and active community of developers. If you encounter issues or need assistance, you can easily find solutions and resources online.
Integration with Other Technologies:Â Python integrates seamlessly with other technologies and libraries, allowing you to combine speech recognition with natural language processing (NLP), machine learning, and more.
Python, with its extensive libraries and user-friendly syntax, is an excellent choice for implementing speech recognition. Python offers various libraries for implementing speech recognition, each with its own set of features and advantages. Some popular choices include:
SpeechRecognition:Â A library that provides simple and easy-to-use interfaces to several speech recognition engines, such as Google Web Speech API, Microsoft Bing Voice Recognition, and more.
PocketSphinx:Â A lightweight speech recognition engine specifically suited for offline applications.
PyAudio:Â A wrapper around the PortAudio library, pyAudio facilitates audio input and output, making it a useful tool for real-time speech recognition.
Wit.ai:Â A platform that allows you to build speech recognition models using machine learning techniques.
Installation
Before diving into implementation, you need to install the selected library. Using pip, the Python package manager, installation is straightforward. For instance, to install the SpeechRecognition library, open your terminal or command prompt and enter the following command:
pip install SpeechRecognition |
Basic Implementation Steps
Now, let's delve into the basic steps of implementing speech recognition in Python.
Import the Library:Â
Begin by importing the speech_recognition module into your Python script:
import speech_recognition as sr |
Create a Recognizer Object:Â
Speech recognition starts with capturing audio. The sr.Recognizer()Â class from the SpeechRecognition library helps you achieve this. It provides methods to record audio from different sources such as a microphone or an audio file.
recognizer = sr.Recognizer() |
Capture Audio:Â
To recognize speech, you need to capture audio. This can be done using a microphone or by providing a path to an audio file:
# Using the microphone with sr.Microphone() as source: Â Â Â Â print("Say something...") Â Â Â Â audio = recognizer.listen(source) # Using an audio file audio_file_path = "sample.wav" with sr.AudioFile(audio_file_path) as source: Â Â Â Â audio = recognizer.record(source) |
Apply Speech Recognition:Â
Now comes the exciting part – applying speech recognition to the captured audio:
try:     text = recognizer.recognize_google(audio) # You can use other engines as well     print("You said:", text) except sr.UnknownValueError:     print("Sorry, could not understand audio.") except sr.RequestError as e:     print("Error connecting to the API:", e) |
Handle Exceptions:Â
Error handling is crucial for a robust application. Handling exceptions, such as UnknownValueError and RequestError, ensures that your program gracefully manages unexpected scenarios. To optimize performance, consider adjusting parameters like sample rate and chunk size based on the requirements of your application.
Advanced Speech Recognition Concepts
While the basic implementation outlined above is a great starting point, there are some advanced concepts and techniques you can explore to enhance your speech recognition implementation.
1. Language and Keywords
The recognize_google()Â function allows you to specify the language and keywords parameter. This is particularly useful when you're expecting speech in a specific language or when you want to recognize particular keywords.
text = recognizer.recognize_google(audio, language="en-US", keywords=["important", "data", "analysis"]) |
2. Adjusting for Ambient Noise
Background noise can affect the accuracy of speech recognition. To mitigate this, you can adjust for ambient noise before capturing the audio:
with sr.Microphone() as source: Â Â Â Â recognizer.adjust_for_ambient_noise(source) Â Â Â Â print("Say something...") Â Â Â Â audio = recognizer.listen(source) |
3. Working with Confidence Levels
Speech recognition engines often provide a confidence level for the transcribed text. You can access this confidence level and use it to filter out low-confidence results:
try: Â Â Â Â result = recognizer.recognize_google(audio, show_all=True) Â Â Â Â for alternative in result["alternative"]: Â Â Â Â Â Â Â Â if alternative["confidence"] > 0.7: Â Â Â Â Â Â Â Â Â Â Â Â print("High confidence:", alternative["transcript"]) except sr.UnknownValueError: Â Â Â Â print("Sorry, could not understand audio.") except sr.RequestError as e: Â Â Â Â print("Error connecting to the API:", e) |
Implementing Speech Recognition with Google Web Speech API
Google provides a powerful Web Speech API that you can use for speech recognition in Python. To use it, you need an internet connection as it relies on Google's online services. Here's how you can use the Google Web Speech API:
import speech_recognition as sr # Initialize the recognizer recognizer = sr.Recognizer() # Use the default microphone as the audio source with sr.Microphone() as source: Â Â Â Â print("Say something...") Â Â Â Â audio_data = recognizer.listen(source) Â Â Â Â Â Â Â Â try: Â Â Â Â Â Â Â Â text = recognizer.recognize_google(audio_data) Â Â Â Â Â Â Â Â print("You said:", text) Â Â Â Â except sr.UnknownValueError: Â Â Â Â Â Â Â Â print("Speech recognition could not understand audio") Â Â Â Â except sr.RequestError as e: Â Â Â Â Â Â Â Â print("Could not request results from Google Web Speech API; {0}".format(e)) |
This code is similar to the previous microphone example, but it uses Google's Web Speech API for recognition.
Building a Voice Assistant
Now that you've learned the basics of speech recognition in Python, you can take it a step further by building your own voice-controlled assistant. Here's a simplified example using the SpeechRecognition library:
import speech_recognition as sr # Initialize the recognizer recognizer = sr.Recognizer() # Function to perform voice commands def voice_assistant():     with sr.Microphone() as source:         print("How can I assist you?")         audio_data = recognizer.listen(source)                 try:             command = recognizer.recognize_google(audio_data)             print("You said:", command)                         # Add your commands and actions here             if "open browser" in command:                 # Code to open a web browser                 pass             elif "play music" in command:                 # Code to play music                 pass             # Add more commands as needed                     except sr.UnknownValueError:             print("Speech recognition could not understand audio")         except sr.RequestError as e:             print("Could not request results from Google Web Speech API; {0}".format(e)) # Call the voice assistant function voice_assistant() |
In this example, we've created a basic voice assistant that listens for commands and takes actions based on the recognized commands. You can expand and customize it to suit your needs.
Handling Recognition Errors
Speech recognition isn't always perfect, and you may encounter recognition errors. To improve accuracy, consider the following tips:
Use a high-quality microphone for better audio input.
Ensure the audio file or microphone input has minimal background noise.
Experiment with different ASR engines or models to find the one that works best for your application.
Implement error handling in your code to gracefully handle recognition failures.
Practical Use Cases
Implementing speech recognition in Python opens the door to a wide range of practical applications. Let's explore a few of them:
1. Voice Assistants
You can create your voice-controlled virtual assistant, similar to Siri or Alexa, that performs tasks based on voice commands.
2. Transcription Services
Build a transcription service that converts recorded audio, such as interviews or meetings, into written text automatically.
3. Language Learning Apps
Develop language learning applications that help users practice pronunciation and receive instant feedback.
4. Accessibility Features
Create applications that assist individuals with disabilities by converting spoken language into text, making communication more accessible.
5. Interactive Games
Design interactive games that respond to voice commands, adding an extra layer of engagement for players.
Future Trends in Speech Recognition:
The field of speech recognition continues to evolve with advancements in machine learning and deep learning. Techniques such as transformer-based models have significantly improved the accuracy of speech recognition systems. Additionally, the integration of speech recognition into various devices and applications indicates a future where human-computer interaction becomes even more seamless. The future of speech recognition holds exciting possibilities:
Enhanced Accuracy:Â Ongoing advancements in machine learning will likely lead to higher accuracy rates, even in noisy environments.
Multilingual Support:Â Speech recognition systems will continue to improve their ability to understand and process various languages.
Integration with AI:Â Integration with artificial intelligence will enable more natural and context-aware interactions.
Final Words
Implementing speech recognition in Python opens up a world of possibilities for creating innovative applications that leverage spoken language. With the SpeechRecognition library and the insights provided in this article, you have the foundation to explore speech recognition further, experiment with different applications, and contribute to the exciting developments in this field.
In conclusion, speech recognition is a dynamic technology that holds immense potential. By following the steps outlined in this article, you can begin your journey into the realm of speech recognition and unlock the power of transforming spoken words into digital text.
Remember, practice and experimentation are key to mastering speech recognition, so feel free to explore, innovate, and create.